Integer Basics
Some examples of basic arithmetic in C.
Convert fahrenheit to celsius
#include <stdio.h>
/* print Fahrenheit-Celsius table
for fahr = 0, 20, ..., 300; floating-point version */
int main() {
printf("FAHRENHEIT -> CELSIUS\n");
float fahr, celsius;
float lower, upper, step;
lower = 0;
upper = 300;
step = 20;
fahr = lower;
while (fahr <= upper) {
celsius = (5.0/9.0) * (fahr - 32.0);
printf("\t %3.0f %6.1f\n", fahr, celsius);
fahr = fahr + step;
}
}
Convert celsius to fahrenheit
#include <stdio.h>
/* print Fahrenheit-Celsius table
for fahr = 0, 20, ..., 300; floating-point version */
#define UPPER 300
#define LOWER 0
#define STEP 20
int main() {
printf("CELSIUS -> FAHRENHEIT\n");
float fahr, celsius;
for (celsius = LOWER; celsius <= UPPER; celsius = celsius + STEP) {
fahr = (celsius * (9.0/5.0)) + 32.0;
printf("\t %3.0f %6.1f\n", celsius, fahr);
}
float thelow = (33 * (9.0/5.0)) + 32.0;
float thehigh = (37 * (9.0/5.0)) + 32.0;
printf("%6.1f %6.1f\n", thelow, thehigh);
}
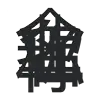