Recursion
Some notes about recursion.
Most Importantly
The most important thing when writing a recursive function is to have a solid base case. AKA, have a solid case for when you EXIT recursion.
package main
import "fmt"
func foo(n int) int {
if n == 1 {
return 1
}
return n + foo(n - 1)
}
func main() {
fmt.Println(foo(5))
}
It is ABUNDANTLY clear here when we stop recursion, when n == 1.
Stages of recursion
There are three stages.
- Pre - before recursion has begun
- Recurse
- Post - when the base case has been met, and we start returning up the call stack
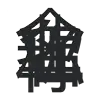