GO CONTEXT
The go programming language standard library ships with a package called context
. This package is basically used for maintaining a, uhm, context in a time-bound lifecycle. A time-bound lifecycle, like, for example, a request/response cycle.
context.Context
can serve as a key/value store over the course of a request lifetime on a server.
Here’s a contrived example.
import (
"context",
"fmt"
)
func enrichContext(ctx context.Context) context.Context {
// set a key/value
ctx.WithValue(ctx, "request-id", "12345")
return ctx
}
func logRequestId(ctx context.Context) {
// access value
rId := ctx.Value("request-id")
fmt.Printf("Request ID: %s", rId)
}
func main() {
// initalize a contex
ctx := context.Background()
ctx = enrichContext(ctx)
logRequestId(ctx)
}
This key/value feature is just the icing on top of the cake. The real use case of context
is for managing timeouts/deadlines, timeboxing request lifecycles, etc.
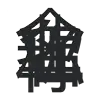