...
) in Go
The Ellipsis (In JavaScript, it means Rest/Spread.
In English, it means you’re a huge bitchface.
In Go, it means these following things.
Variadic Function Argument
Sometimes functions can take a variable number of arguments, all of the same type. For example, fmt.Printf
is a variadic function — it can take any number of arguments from 1 to hundreds(no idea the maximum).
func sum(nums ...int) int {
s := 0
for _, n := range nums {
s += n
}
return s
}
Like a JavaScript rest operator.
Passing Values to Variadic Functions
Similarly, we can spread a slice into a variadic function call using an ellipsis, which will then behave as if we were calling it with multiple arguments.
primes := []int{2, 3, 5, 7}
fmt.Println(Sum(primes...)) // 17
Array Literals
Totally different use case!! Not like rest/spread. But also a kind of rare case, because in practice we don’t end up using arrays all that much.
We can use an ellipsis when declaring an array literal to let the compiler know the length of the array. It’s like the typedef saying, “Don’t ask me, just look at the length of the array literal!”
blink182 := [...]string{"Tom", "Marc", "Travis"}
fmt.Println(len(blink182))// 3
The Go Command
The last use case is not a syntax/language feature, but a feature of the go tool. You can use an ellipsis as a wildcard when using the go tool when describing package lists.
This command formats all packages in the current directory and its subdirectories.
go fmt ./...
That’s it! Four use cases.
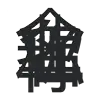