Interface vs. Struct
For some reason, I always get confused about this. They don’t actually have that more overlap.
Struct
Structs are basically like strongly-typed object literals.
type Car struct {
Make string
Model string
GasCapacity int
}
func (c Car) Drive() {
fmt.Printf("Driving %s %s", c.Make, c.Model)
c.GasCapacity--
return
}
func main() {
car := &Car{
Make: "Volvo",
Model: "740 turbo intercooler",
GasCapacity: 8,
}
car.Drive()
}
Interface
Interface is like an abstract type. You can’t instantiate an interface. It’s just a protocol, defining a behavior, but provides no implementation.
// interface for some thing that can be driven
type Driver interface {
Drive()
}
type Car struct {
Make string
Model string
GasCapacity int
}
func (c Car) Drive() {
fmt.Printf("Driving %s %s", c.Make, c.Model)
c.GasCapacity--
return
}
type Moto struct {
speed int
}
func (m Moto) Drive() {
fmt.Printf("Driving moto")
}
func printDrivableVehicles(vehicles []Driver) {
for _, v := range vehicles {
v.Drive()
fmt.Println("Just drove something")
}
}
func main() {
car := Car{
Make: "Volvo",
Model: "740 turbo intercooler",
GasCapacity: 8,
}
moto := &Moto{
speed: 1000,
}
mytoys := []Driver{car, moto}
printDrivableVehicles(mytoys)
}
This is like saying “you can only satisfy me if you have a function called “drive” on you. Bit of a convoluted example but the point is made.
Structs can implement interfaces, but this is purely implicit. There is no implements
keyword in Go. By implementing the function names, the struct implicitly satisfies the interface.
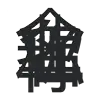