The range
keyword in go is used to turn something into “an iterable”. Calling it an “iterable” is my invention. Anyways, it’s used to turn a variety of data structures into something you can loop over.
A simple example
package main
import "fmt"
func main() {
nums := []int{2, 3, 4}
sum := 0
// loop over a slice of numbers
for _, num := range nums {
sum += num
}
fmt.Println("sum:", sum)
// loop over a map
kvs := map[string]string{"a": "apple", "b": "banana"}
for k, v := range kvs {
fmt.Printf("%s -> %s\n", k, v)
}
//loop over a string
for i, c := range "go" {
fmt.Println(i, c)
}
}
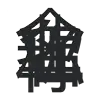