Collections
It’s not simple like JavaScript. There are a bunch of different ways to do collections of objects in Java. The basic Array
in Java has a set length once instantiated, and cannot change. There are a bunch of things in java.util.*
for managing collections and lists of things instead.
The very base of them all is the Collection
class. The Collection
is basically a high-level interface providing guideline for how to implement a collection of stuff, making different data structures relatively predictable and uniform — they all have a common set of methods, like add
, remove
and whatnot.
The ArrayList
- The most basic collection, an array that automatically expands itself.
public class ApplesAndOrangesWithoutGenerics {
@SuppressWarnings("unchecked")
public static void main(String[] args) {
ArrayList apples = new ArrayList();
for(int i = 0; i < 3; i++) {
apples.add(new Apple());
}
// No problem adding an Orange to apples: apples.add(new Orange());
for(Object apple : apples) {
((Apple) apple).id();
// Orange is detected only at run time
}
}
}
There are also these:
Set
HashSet
Map
HashMap
ArrayList
LinkedList
Stack
Queue
PriorityQueue
Autoboxing
Collections can’t hold primitives, only objects. Autoboxing is a term that refers to a nice thing that happens when you make a collection of primitives — it converts it to a wrapper object for you on the way in and out.
Iterator
In addition to the Collection
interface, there is also the Iterator
interface. This is an interface that all Collections implement as a nested/related object, used as a cursor to a given member/item in the collection. You can ask a collection to hand you an iterator by calling .iterator()
on the collection. It returns the first item in the collection. You can then call .next()
on the iterator, and it will return you the next item. Iterator
also has other methods, like .hasNext()
, .prev()
, .remove()
, etc.
Use this to move through (iterate through) a collection. More here -> docs.
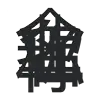