Java Interfaces
In addition to the heavy use of class-based inheritance, Java also offers type interfaces as a way to compose and type your application code. There are also abstract classes, which act in a similar way.
Abstract Classes
This is what an abstract class looks like.
abstract class Basic {
abstract void unimplemented();// abstract method
int f() { return 111; } // default base method
}
This is how to use it.
public class Instantiable extends Basic {
@Override void unimplemented() {
System.out.println("now it is implemented");
}
public static void main(String[] args) {
Basic ui = new Instantiable();
}
}
Abstract classes are mainly useful for telling the user or the compiler that a class is explicitly a base class, and cannot be instantiated. They also, of course, are helpful for inheritance OOP shit.
Interfaces
On the other hand, there are interfaces. Interfaces are better, and more readable in my opinion. They tell the user how to implement a classes API, while allowing for more flexibility and readability.
They look like this:
interface Concept { // Package access
void idea1();
void idea2();
default void defaultIdea() {
System.out.println("Default idea!");
}
}
class Reality implements Concept {
@Override public void idea1() {
System.out.println("idea1");
}
@Override
public void idea2() {
System.out.println("idea2");
}
public static void main(String[] args) {
Concept instance = new Reality();
instance.idea1();
instance.idea2();
instance.defaultIdea();
}
}
One noteable difference to TypeScript, for example, is the use of default methods on interfaces. Methods defined (and implemented) on an interface are fully available for execution on a class which implements that interface.
Multiple Inheritance
This is total madness, but Java can inherit from multiple classes or interfaces at once. It looks like this:
interface Sam1 {
default void sam() {
System.out.println("Sam1::sam");
}
}
interface Sam2 {
default void sam(int i) {
System.out.println(i * 2);
}
}
class Sam implements Sam1, Sam2 {}
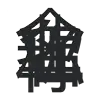