Java Basics
Most of these notes are coming from On Java 8 by Bruce Eckel.
Everything is an Object
If there’s one thing that you really can’t escape in Java, it’s that it is hardcore Object Oriented Programming (OOP).
Everything in Java inherits from the base class of Object. Even numbers and strings.
Everything is essentially class-based, and you can’t really do “scripting” without instiating some classes.
For example, string:
String s = new String("asdf");
This is how you instantiate a string. You can, of course, instantiate with a string literal also:
String s = "asdf"
All Variables Are References
In Java, variabels are references. It’s actually similar to JavaScript in this regard — if you store an object into a variable in JavaScript, it’s a reference to the original object. Mutatiting the variable will also mutate the object.
The same is true in Java.
class Tank {
int level;
}
public class Assignment {
public static void main(String[] args) {
Tank t1 = new Tank();
Tank t2 = new Tank();
t1.level = 9;
t2.level = 47;
t1 = t2;
t2.level = 27;
System.out.println("t1 level: " + t1.level);
}
}
In this case, we’d see: "t1 level: 27"
Primitives
- boolean
- char
- byte
- short
- int
- long
- float
- doubl
- void
Type Casting
You can type cast in Java using this syntax
public class Stringer {
public static void main(String[] args) {
char s = '0';
long i = (long)s;
System.out.print(i);
}
}
A bit about the Java Runtime
Of course the key component to the Java runtime here is the JVM (Java Virtual Machine) which I still know basically nothing about. However, there are some things that are noteworthy about how Java works at runtime, particularly where stuff is stored at runtime.
- Registers
- The Stack
- The Heap
Java Files and your program
There are a few gotchas here.
- It’s best to name the file the same name as your main public class in the file, e.g., class
Demolisher
should live inDemolisher.java
- Similar to C, Java can function in a really similar way using a
main
function as the entry point to a program. - Package namespaces follow the convention of your reversed domain, e.g.,
johnny.com
’s package system/library would start withcom.johnny
- The file structure of your Java program must reflect exactly the namespace hierarchy of your Java program, resulting in often deep nesting of the files in your java program.
- You can import all classes of a library using star, e.g.,
import com.johnny.*
Java Programs. What are they?
Java runs programs on the jvm
— Java Virtual Machine. You compile your program and then run it on any platform inside the jvm
. When you compile your program, it outputs into a bunch of files, not just a static binary. Those files can be in a folder, or compressed and put into a .jar
file. It’s a Java Archive.
Instead of creating a single static binary, a compiled Java program consists of a bunch of .class
files. Each class in your program compiles to a .class
file. Inside of these files is Java byte code, to be interpreted by the Java runtime.
In this regard, one noteable thing about Java is that some things happen at compile time, some things happen on-the-fly at runtime. Some examples of compile time things would be: some static type checking, and some compilation of class methods. For example, class methods which are labeled with the static
keyword will only be compiled once, whereas some other class methods will actually be composed at execution time by the Java runtime.
Formatting, Style, and the Language
- Java uses semicolons. They are required.
- Use PascalCase for file names and class names.
- Use camelCase for variables.
- Type definitions are required. There is type casting and some type coercion stuff, but no type inference.
- Java is very into “call signatures”. Meaning the return type, arguments + argument types are very important.
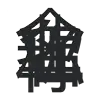