Functions. In Python.
Functions are created with the def
keyword in Python. There are also some interesting things about functions in Python.
Named and Positional Arguments
Kind of like Ruby, you can use named arguments (aka keyword arguments) to call a function. Like this:
def order_lunch(name, food="sandwich", price=0):
print("Hi i'm", name, "I would like", food, "here is" price, "dollars")
In this function, the only required argument is name
. However, you can call this function with only name
and price
defined if needed, and let the function use food
’s default argument, like this:
order_lunch("Johnny", price=200)
Restricting to Positional-only or Keyword-only
As mentioned, we can use keyword arguments in python functions. The default behavior is for keyword-based arguments to be optional, i.e., you can use them if you want, can also not use them.
We can also restrict a python function signature to explicitly require keyword-based arguments (and thus not allowing it to be called with position-based arguments). Similarly, we can restrict a python function signature to disallow keyword-based arguments, or any combination of the two.
This is done with the use of some special symbols in the function declaration — anything preceding /
becomes position-only, and anything following *
becomes keyword-only.
def pos_only_arg(arg, /):
print(arg)
def kwd_only_arg(*, arg):
print(arg)
def combined_example(pos_only, /, standard, *, kwd_only):
print(pos_only, standard, kwd_only)
*args
Python has a feature similar to JavaScript’s rest operator, the ...args
in JS-world. In Python, it’s an argument declared with an *
in front of it.
def list_people(*people, gender="male"):
print(", ".join(people), "are all", gender)
Similarly, you can unpack a list into a function call using the same syntax when you call the function.
the_bros = ["deng xiaoping", "jiang zemin", "hu jintao"]
list_people(*the_bros)
the_missuses = ["fan bingbing", "peng shuai"]
list_people(*the_missuses, "female")
In the same fashion, dicts can be totally unpacked to fulfill a python func signature by using all they keys to serve as the keyword arguments with the **
operator:
def list_person_attrs(height, name, pronouns):
print(name, "is", height, "cm tall and uses the ", "/".join(pronouns), "pronouns")
me = {"height": 179, "name": "Johnny", "pronouns": ("he", "him")}
list_person_attrs(**me)
Docstrings
A strong python convention is to use something called “docstrings” to comment functions. These are used in some documentation-generation programs, but also just as a common readability convention for function defs.
def check_sanity():
"""This function checks sanity of the universe"""
return 1 + 1 == 2
Read more about the conventions for docstrings here.
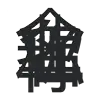