Syntax interesting.
I was reading python org’s doc on control flow when I realized Python does indeed have some interesting syntax oddities.
else
You can use the else
clause in places other than if/else statements. For example, a loop:
for n in range(2, 10):
for x in range(2, n):
if n % x == 0:
print(n, 'equals', x, '*', n//x)
break
else:
# loop fell through without finding a factor
print(n, 'is a prime number')
or a try/catch clause:
def do_impossible_math:
n = 0
try:
n = 1/0
finally:
n = 42
return n
pass
Python has a built-in global noop, called simply pass
.
# empty class
class MyEmptyClass:
pass
# waits for keyboard interrupt
while True:
pass
None
Similarly, python has a nice little keyword called simply None
, which is basically just null
or nil
.
x = None
print(x)
with
Python has a weird keyword called with
. The only thing I can think of that is anything remotely similar is Go’s defer
. with
works by calling the __exit__
method automatically on an object which is scoped in a fail-safe block. That’s confusing, I know. Here’s a classic example:
print("We're gonna check the secret message")
with open("secret.txt", "r") as file:
print(file.read())
print("The file has been closed")
When we use with
, it handles closing the file for us, automatically. Even if it fails to open the file. In other words, with
makes it easy to write code that requires some cleandown task regardless of if the block succeeded or failed. Kind of like a try/finally block.
List Comprehensions
Python’s “array”-like data structure is known as list
. There’s nothing too curious about the list
in Python, it supports the usual stuff such as .append
, .pop
, .reverse
, etc.
lucky_nums = [911, 888, 8, 69, 311, 420 ]
nsfw_lucky_nums = [420, 69]
sfw_nums = []
for x in lucky_nums:
if x not in nsfw_lucky_nums:
sfw_nums.append(x)
print(sfw_nums) # [911, 888, 8, 311]
# Python also allows us to write this as an inline statement, for extra codecleanliness™️.
sfw_nums = [x for x in lucky_nums if x not in nsfw_lucky_nums]
print(sfw_nums) # [911, 888, 8, 311]
Python also supports the same syntax for sets.
Also, another cool example of list comprehensions: using them for inline for loops to do a map-like effect.
# let's just assume every item in rows has an "id" property
def list_to_dict(rows: list):
return {row["id"]: row for row in rows}
print(list_to_dict([{"id": 1, "val": "yesss"}, {"id": 2, "val": "nooo"}]))
Python also supports the same syntax for lists.
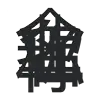