React Native
Notes on React Native.
Tutorials
- React Native Tutorial for Beginners - Build a React Native App - Concise free tutorial with the basics of the dev environment.
React Native CLI vs. Expo
There are two primary options when setting up a new React Native project: expo and React Native CLI. Expo is a nice meta-framework for creating React Native apps, and it provides a lot of out-of-the-box configurations already taken care of, for example: bundling, distribution, light integration with Android and iOS emulators, and a nice CLI.
I’m not sure what the advantages are for React Native CLI in-the-raw, I’m sure as time goes on I will eventually choose to use raw React Native CLI.
Screen Resolution Stuff
When working in React Native, you come across code like this for styling:
const styles = StyleSheet.create({
width: 20,
height: 20,
})
What does 20
here mean? Pixels?
In fact, it’s device independent pixels, aka DIPS. Basically, it’s a unit that compensates for screen resolution of different devices, and we can assume they look “almost the same” across devices.
Debugging
Debugging in react native is a bit trickier.
Standalone Debuggers
So far, I’m trying Reactotron. It’s useful for both React Native and web React projects, but kind of limited. Basically like a little debugger. It’s also one of the best ways to see network traffic.
There’s also React Native Debugger which is said to have good network debugging.
Debugging in the iOS Simulator
You can pop a developer menu in the iOS simulator by pressing command + d
while focused on the screen. From here, you can choose inspect
. Not sure how this works yet.
However, there is one good debugging tool available from the iOS simulator debug screen.
- Press
command + d
- Choose
debug
- Open http://localhost:8081/debugger-ui/ in Chrome
This should open a little debugging UI in your browser powered by WebRTC. Open the console log. Console.logs here are the console.logs from your RN app. Allegedly the network tab should also show network I/O from the app. Haven’t gotten this to work yet.
Note: the iOS debugger connected to chrome is heeeavy. Will be slow and consume lots of battery. Turn it off when you’re not using it.
Debugging in VS code
- Install
React Native Tools
from VS Code extensions marketplace. It’s the one from Microsoft. - With the React Native codebase open in VS Code, choose the debug tab (
command + shift + d
) - From here, it will prompt you to configure a
launch.json
file. Do that. - Choose React Native from the dropdown
- You can add items to the
launch.json
file, or you can choose some of the pre-configured debug flows from the dropdown menu next to the green arrow. - From here, you can drop breakpoints in your code and step through functions while inside vs code.
Debugging and developing with Android Studio
The dev workflow for android devices is a bit finicky as well. If there’s a key theme here with React Native, it’s finicky.
Basically the steps are:
- Install android studio
- After it’s installed, go to preferences (
command + ,
in the app), - appearance and behavior -> system settings -> android SDK
- Under SDK Platforms tab, make sure the latest version of android is installed, and any other versions of android that you want to test/dev against
- For each version of android that you installed, make sure you have at least the SDK and the “system image” checkboxes checked.
- Under the SDK Tools tab, make sure you have both Android Emulator and Android SDK Platform-tools checked
- Press OK, wait forever for everything to install
- After it’s done, add this to your bash/zsh/fish profile:
# android emulator + android studio
export ANDROID_SDK_ROOT=$HOME/Library/Android/sdk
export PATH=$PATH:$ANDROID_SDK_ROOT/emulator
export PATH=$PATH:$ANDROID_SDK_ROOT/platform-tools
The emulator
command should now be in your PATH. LASTLY, make sure you create a virtual device in Android Studio. To do this:
- From main Android Studio welcome page, choose the vertical
...
in the upper right hand corner, then chooseVirtual Device Manager
- Add a virtual android device
- That should be it.
Resources
- Solito - unified navigation library for RN and Next.js. Opinionated arch, monorepo FE and BE and RN arch provided/boilerplate.
- Ignite - react native boilerplate
- tamgui - cross platform apps meta framework (web + react native).
- restyle - library for styling react native UI components, inspired by system UI
- expo - really nice bundler + react native toolchain.
- some boilerplate
- belt - a tool for starting react native apps
Codebases
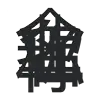