Rust Conventions And Such
Code Formatting
In rust, we use both snake_case and PascalCase. They serve different purposes.
- Variables and function names are typically snake case
- Module names are typically use snake_case
- Struct, enums, and trait names are typically written in PascaleCase.
- SCREAMING_SNAKE_CASE is used for constants and static variables.
Deriving Traits
This is commonly seen in rust codebases. Use #[derive]
to automatically implement traits like Clone
, Debug
, PartialEq
, etc., for your types.
#[derive(Debug, Clone, PartialEq)]
struct MyStruct {
field1: i32,
field2: String,
}
Breakdown:
derive
is a macro for composing traitsDebug
is used for formatting a value using the{:?}
formatter inprintln!
and similar macrosPartialEq
trait allows for the comparison of objects for equality using the==
and!=
operators. AKA, allows comparing fields for value not just identity.Clone
trait allows for the explicit duplication of an object. When you add this trait, the struct automatically gets aclone
method that creates a deep copy of the instance.
Clippy
clippy
is a commonly used rust linter. Use it.
cargo clippy
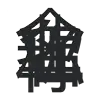