Other Interesting Rust Syntax
Miscellaneous findings.
Return values
One thing I found strange about rust is the way values are returned from functions. You can just use a return
keyword like most langs, but one unique thing about rust is that a semicolon can be the difference between an implicit return and a simple “value invocation” which does not return anything.
Take these two examples:
pub fn doStuffAndReturnIt() {
// do stuff
let x = 5;
x
}
pub fn doStuffAndThenDoNothing() {
// do stuff
let x = 5;
x;
}
In Rust, if the last expression in a function has no semicolon, it becomes the return value. So in the first example, we return x
, in the second example, we return ()
(the unit type) and the value of x
is discarded.
mut
In rust, variables are immutable by default. Make a mutable variable with the mut
keyword.
let x = 5; // immutable
let mut y = 10; // mutable
!
on stuff
In rust, macros can be used in a c-esque way, or they can be invoked while hidden as normal functions by using a !
and calling it like a function. That’s a bad sentence. Here’s a better example:
println!("Hello, World!"); // This is a macro
Other examples of common macros:
- vec![]: Creates a vector.
- format!(): Creates a formatted string.
- panic!(): Triggers a panic (terminating the program).
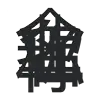