Pattern Matching in Rust
Rust has a really awesome feature called Pattern Matching. Elixir also has this. In Rust, it’s used for matching complex data structures in a clean and expressive way. It looks like this:
match value {
pattern1 => result1,
pattern2 => result2,
_ => fallback_result, // The `_` is a catch-all pattern
}
Note the use of _
as the catch all.
Pattern Guards
We can also use if
statements within pattern matching statements to do something called “pattern guards”. Looks like this:
let num = 7;
match num {
x if x % 2 == 0 => println!("Even number"),
x => println!("Odd number"),
}
Destructuring
We can also destructure the input data type, kinda similar to Javascript destructuring.
// example with a tuple
let point = (3, 7);
match point {
(x, y) => println!("x is {} and y is {}", x, y),
}
// example with an inline struct
let p = Point { x: 5, y: 10 };
match p {
Point { x, y } => println!("x is {} and y is {}", x, y),
}
This and pattern guards allow us to get even more granular and specific with how we conditionalize/match the data.
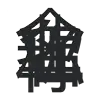