struct
This keyword is the most commonly used way to define datatypes in rust. Like an interface in typescript, or a struct in Go.
struct Car {
x: i32,
y: i32,
brand: String,
}
impl
Similar to how you can add functions to a struct in Go, you can implement methods on a struct in Rust. Like this:
struct Car {
x: i32,
y: i32,
brand: String,
}
impl Car {
fn new(x: i32, y: i32, brand: String) -> Self {
Self { x, y, brand }
}
fn drive(&mut self, distance: i32) {
println!("driving: {} miles", distance);
self.x += distance;
}
}
fn main() {
// create a new car
let mut volvo = Car::new(0, 0, String::from("volvo"));
// drive the car
volvo.drive(10);
}
Other Notes
- Associated functions are kind of like class methods and associated constants are kind of like class members/enums. These are accessed with
::
, e.g.,Car::new()
- Calling stuff on the instances of a rust struct is done with normal dot notation e.g.,
volvo.drive()
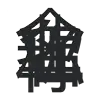