trait
Traits in Rust are kind of like interfaces in other languages, except they only define methods, no fields of e.g., strings, ints, etc. It’s not a data structure/object.
Traits define a behavior.
The naming convention for traits: it should be a verb, and in PascalCase. Examples: Display
, Debug
, Clone
.
You also use the impl
keyword to implement a trait.`
trait Drivable {
fn left(self);
fn right(self);
fn forward(self);
fn backward(self);
}
struct Car {
make: String,
mileage: i32,
x: i32,
y: i32,
}
impl Car {
pub fn new(make: String) -> Self {
Car {
make,
x: 0,
y: 0,
mileage: 0,
}
}
}
impl Drivable for Car {
fn left(mut self) {
self.x -= 1;
}
fn right(mut self) {
self.x += 1;
}
fn forward(mut self) {
self.y += 1;
}
fn backward(mut self) {
self.y -= 1;
}
}
let volvo = car::Car::new(String::from("volvo"));
volvo.forward();
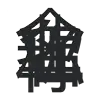