Vec
and VecDeque
Arrays in rust, similar to Go, are actually very primitive things. Arrays have a known size that can’t be changed. They look like this:
let arr: [i32; 3] = [1, 2, 3]; // A fixed-size array of 3 integers
So, in practice, for list-y stuff we don’t use arrays. We use Vec
s, or vectors. These are growable, heap-allocated arrays, good for dynamic stuff like how we’d use arrays in JS or elsewhere. They are also typed, only allowing you to store stuff of type T
.
let mut numbers = Vec::new(); // Create an empty vector
numbers.push(1); // Add elements
numbers.push(2);
numbers.push(3);
println!("{:?}", numbers);// Output: [1, 2, 3]
numbers.pop(); // Remove the last element
let guy = numbers.get(1);
println!(guy); // 2
println!("{:?}", numbers); // Output: [1, 2]
You can also create an inline vec on the fly like this:
let numbers = vec![1, 2, 3]; // Create a vector with initial values
println!("{:?}", numbers); // Output: [1, 2, 3]
VecDeque
?
What about Rust, being the borderline overengineered megalang that it is, also provides a ring buffer implementation as part of the std lib. Which is cool!
That’s basically what VecDeque
is — a double-ended array/list which is implemented as a ring buffer. Double-ended meaning both adding and removing items is quite efficient.
use std::collections::VecDeque;
fn main() {
let mut deque: VecDeque<i32> = VecDeque::new();
deque.push_back(1); // Add to the back
deque.push_front(0); // Add to the front
println!("{:?}", deque); // Output: [0, 1]
deque.pop_front(); // Remove from the front
println!("{:?}", deque); // Output: [1]
}
The VecDeque
is slightly more efficient if you need a list for adding and removing from both front and back of the list. Other than that, it’s pretty much the same as Vec
.
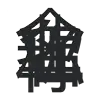