Variables + Shadowing
In Rust, variables are immutable by default. Rust really sets the tone, letting you know the compiler is going to be strict about mutability and the share of data between parts of your code.
Rust DOES let you create mutable variables, like this:
fn main() {
let mut x = 5;
println!("The value of x is: {x}");
x = 6;
println!("The value of x is: {x}");
}
We changed the value of x
.
That’s mutability in rust.
Shadowing
Shadowing is different than using mut
. You can declare a new variable with the same name as a previous variable. In Rust-speak, we say that the first variable is shadowed by the second.
Unlike mut
, shadowing lets us change the type of the value but reuse the same name:
fn main() {
let spaces = " ";
let spaces = spaces.len(); // spaces is now a number, not a string
println!("Number of spaces: {spaces}"); // Will print 3
}
Both of these variables (spaces
as a string and spaces
as a number) are immutable.
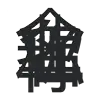