Shader Vectors
Just a reminder: a vector is an array of scalar values of a set length.
In shaders, and specifically GLSL flavor of shaders, these can be vec2
, vec3
or vec4
.
vec2
is two values.
vec2 coords = vec2(0.21,0.3);
vec3
is three values.
vec3 color = vec3(0.1, 0.45, 0.2);
vec4
is four values. Also, just a reminder: you can also “fold” or spread vectors into vectors of greater length. For example, folding a 3-value RGB value into an RGBA vec4
value:
vec3 color = vec3(0.1, 0.45, 0.2);
gl_FragColor = vec4(color,1.0);
Dot notation alias
Another cool important thing to note about vec
types in shaders: because they are so commonly used for coordinates, RGB(A) values and similar, there are built-in aliases to the members of a vector that make it easy to treat them as C-like structs instead of simply lists of floats. For example
vec2 coords = vec2(1.0, 0.9);
// accessing `.x` and `.y`
x = vec2.x;
y = vec2.y
vec3 color = vec3(0.1, 0.4, 0.9);
// accessing RGB values directly
r = vec3.r;
g = vec3.g;
b = vec3.b;
Makes things easy. Also, this example shows how all these values/ways of accessing the value on a vector are interchangeable:
vec4 vector;
vector[0] = vector.r = vector.x = vector.s;
vector[1] = vector.g = vector.y = vector.t;
vector[2] = vector.b = vector.z = vector.p;
vector[3] = vector.a = vector.w = vector.q;
Swizzling
We can actually go totally crazy with these dot notation members and combine them however we want. This is called swizzling:
vec3 yellow, magenta, green;
// Making Yellow
yellow.rg = vec2(1.0); // Assigning 1. to red and green channels
yellow[2] = 0.0; // Assigning 0. to blue channel
// Making Magenta
magenta = yellow.rbg; // Assign the channels with green and blue swapped
// Making Green
green.rgb = yellow.bgb; // Assign the blue channel of Yellow (0) to red and blue channels
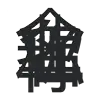