Classes in Swift
Classes behave mostly the same as classes in JS or Ruby.
- Use
class
keyword - Assign class properties within the block using
let
orvar
- Use
init
for initialize,deinit
for any cleanup - Use
func
keyword for class methods - Use
self
to access class instances - Use the
override
keyword to override methods on the parent class
class Car {
let name: String
let year: Double
var mpg: Float
init(name: String, year: Double, mpg: Float) {
self.name = name
self.year = year
self.mpg = mpg
}
func simpleDescription() -> String {
return "This car mpg is \(self.mpg) miles per gallon"
}
}
let volvo = Car(name: "xc60", year: 2023, mpg: 31.2)
print(volvo.name)
volvo.mpg = 30.0
print(volvo.simpleDescription())
You can also do OOP-ish class inheritence, by using the :
operator, like a typedef.
class Vehicle {
let wheels: Double
init(wheels: Double) {
self.wheels = wheels
}
func simpleDescription() -> String {
return "This is a vehicle with \(self.wheels) wheels on it"
}
}
class Car: Vehicle {
let name: String
let year: Double
var mpg: Float
init(name: String, year: Double, mpg: Float) {
self.name = name
self.year = year
self.mpg = mpg
super.init(wheels: 4)
}
override func simpleDescription() -> String {
return "This car mpg is \(self.mpg) miles per gallon"
}
}
let volvo = Car(name: "xc60", year: 2023, mpg: 31.2)
print(volvo.name)
volvo.mpg = 30.0
print(volvo.simpleDescription())
Other things about classes:
- Class properties have getters and setters, as well as
willSet
andwillGet
hooks-like things. - Class properties can be
public
andprivate
, andstatic
. Same same.
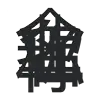