ELIXIR notes
Elixir is a functional programming language created by a former Ruby on Rails contributor named José Valim. It’s a dynamic language which runtime-compiles down to byte code which runs on the erlang Beam VM. Since it runs on the Beam, Elixir is kind of an extension of the Erlang ecosystem — it benefits from the fault tolerant, hyper-concurrent capabilities of Erlang, and also has a nice story for Erlang module interop.
Like Ruby, elixir is also dynamically typed, which means type-checking doesn’t happen during a build step. Types get checked at runtime.
Elixir is very fast.
The syntax is quite similar to Ruby, but in a functional paradigm.
Modules
Elixir is a functional-programming language and requires all named functions to be defined in a module. The defmodule keyword is used to define a module. All modules are available to all other modules at runtime and do not require an access modifier to make them visible to other parts of the program. A module is analogous to a class in other programming languages.
defmodule Calculator do
# ...
end
Naming Conventions
- Module names should use PascalCase.
- A module name must start with an uppercase letter A-Z and can contain letters a-zA-Z, numbers 0-9, and underscores _.
- Variable and function names should use snake_case.
- A variable or function name must start with a lowercase letter a-z or an underscore _, can contain letters a-zA-Z, numbers 0-9, and underscores _, and might end with a question mark ? or an exclamation mark !.
Standard lib
Elixir has a very robust standard lib, which can be browsed here.
Most primitive data types have a strong standard lib module to go along with them, e.g., String
module for common string stuff, Integer
module for common integer stuff.
There’s also a really nice (allegedly!) module called Kernel
, which provides the basic capabilities on top of which the rest of the standard library is built, like arithmetic operators, control-flow macros, etc. This module is globally available, you don’t need to import it.
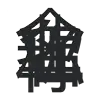