Elixir Functions
Named functions in elixir must be defined in a module. Like ruby, elixir uses the def
keyword.
defmodule Calculator do
def add(x, y) do
x + y
end
end
The last line in a function is the return value — it’s implicitly returned, no return
keyword.
Invoking a function is done by specifying its module and function name and passing arguments for each of the function’s arguments.
sum = Calculator.add(1, 2)
# => 3
Private functions
As mentioned, modules in elixir are kind of analogous to classes in other languages. Like classes having private methodcs, modules can also have private functons. These can be defined using the defp
keyword (instead of def
) and invoked within the same module.
defmodule Calculator do
def subtract(x, y) do
private_subtract(x, y)
end
defp private_subtract(x, y), do: x - y
end
Arity of functions
It is common to refer to functions with their arity. The arity of a function is the number of arguments it accepts. You see this written like subtract/2
- referring to the subtract
function above which takes 2 arguments.
Anonymous Functions
In addition to named functions under a module, elixir also supports anonymous/inline functions. Kinda like lambdas in ruby. They look like this:
add_one = fn (n) ->
n + 1
end
# also can do
add_one = fn (n) -> n + 1 end
# SHORTHAND notation with & operator
add_one = &(&1 + 1)
add_one.(2)
The last one, the shorthand notation, is a bit confusing at first. The &
operator here is serving two purposes: function declaration and argument access. The first one is like saying fn () ->
, the second one (the &1
) is accessing the first argument. If there are two arguments, then there are both &1
and &2
.
You can invoke anonymous functions by calling it like name.()
.
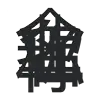